I've been working on a small "Word Finder" game, mostly as exercise to learn CoffeeScript, a language that compiles down to JavaScript. CoffeeScript hides the tricky parts of JavaScript, and brings to the programmer the conventions, idioms and conveniences of modern languages like Ruby and Python. I had just finished taking a course using Python, and I was eager to continue using the style presented by that language.
However, I was confused by how you go about "sharing the code" that you write in CoffeeScript with, say, another piece of JavaScript that a web page might use. In the code that I was writing, I had defined a class, and created an object of that class. I now wanted to use that class in another JavaScript file, but when I referenced that object's variable, it said it was not defined.
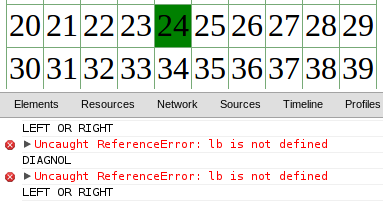
Digging into this, I read on the CoffeeScript website that all code in a CoffeeScript file is hidden in an anonymous function. This makes it "exceedingly difficult to pollute the global namespace by accident." But what if I now wanted to make an object available to the global namespace? What if I wanted to share out some parts of my CoffeeScript code to another JavaScript file?
The CoffeeScript website says "If you'd like to create top-level variables for other scripts to use, attach them as properties on window, or on the exports object in CommonJS." Let me try to break this down, taking the CommonJS suggestion first.
Spending a few minutes learning about CommonJS introduced me to the heady world of server-side JavaScript, specifically NodeJS. (By way of confession, I'm a newcomer to modern front-end development.) Writing modular JavaScript for NodeJS required solutions for importing ("requires") and exporting modules. These solutions for the server eventually made their way back to the client (i.e. the browser), like RequireJS.
Learning these solutions, and retro-fitting my little game to use a modern framework, is something that I knew I'd have to embrace. However, I wanted to explore how to attach variables "as properties on window." It turns out to be a "questionable" practice, the end-result being another global variable, but the actual code to add a window property was deceptively easy:
window.lb = lb
Once I had this line, my other JavaScript could reference the variable lb, and all of its functions and variables.
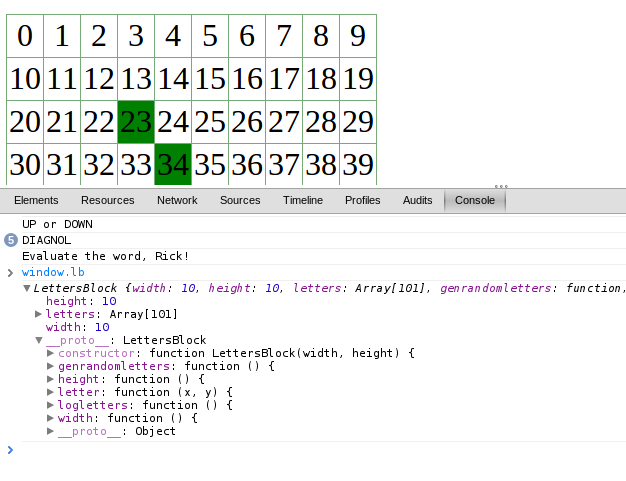
As I mentioned, I do plan to adopt a more modern JavaScript framework, but this gets me going right now, and I'll take that.